Are you tired of spending countless hours on repetitive tasks that drain your productivity? Automate the Boring Stuff with Python is your ultimate solution to streamline mundane processes, giving you more time to focus on what truly matters. This practical programming guide empowers beginners and enthusiasts alike to harness the power of Python to automate everyday tasks, from organizing files to sending emails and beyond.
Python, a versatile and beginner-friendly programming language, has become a favorite among developers for its simplicity and efficiency. With "Automate the Boring Stuff with Python," anyone—regardless of prior coding experience—can learn to write scripts that handle tedious chores. Whether you're a student, a professional, or someone just curious about coding, this book opens the door to a world where you can automate repetitive workflows, saving time and reducing errors.
In this comprehensive article, we'll dive deep into the key concepts, practical applications, and step-by-step guides found in Automate the Boring Stuff with Python. From understanding the basics of Python to exploring advanced automation techniques, this resource-packed guide will equip you with the knowledge and tools to revolutionize the way you work. Let's embark on a journey to transform your daily grind into an efficient, automated process!
Read also:Frankfurt Airport Am Main The Hub Of International Travel
Table of Contents
- What is Automate the Boring Stuff with Python?
- Why is Python Perfect for Automation?
- How to Get Started with Python?
- Essential Python Concepts for Automation
- What Tasks Can You Automate with Python?
- Step-by-Step Guide to Automating Tasks
- Automate File Management Tasks
- How to Automate Web Scraping Tasks?
- Email Automation with Python
- Automate Data Entry and Analysis
- Advanced Tips for Python Automation
- Common Errors and How to Fix Them
- Is Automate the Boring Stuff with Python Right for You?
- FAQs About Automate the Boring Stuff with Python
- Conclusion
What is Automate the Boring Stuff with Python?
"Automate the Boring Stuff with Python" is a hands-on guide written by Al Sweigart, designed to teach readers how to use Python to automate repetitive tasks. This book is tailored for beginners, making complex programming concepts approachable and easy to understand. It covers a wide array of topics, including file manipulation, web scraping, data analysis, and more, all presented through practical examples and exercises.
The book's primary goal is to enable readers to write Python scripts that can handle tedious, time-consuming tasks, such as:
- Renaming or organizing files and folders
- Extracting data from websites (web scraping)
- Automating email responses
- Processing spreadsheets and PDFs
- Performing repetitive data entry tasks
With its straightforward approach and real-world applications, Automate the Boring Stuff with Python has become a go-to resource for anyone looking to enhance their productivity through automation.
Why is Python Perfect for Automation?
Python is highly regarded as one of the best programming languages for automation, and there's a good reason for that. Its simplicity, readability, and extensive library support make it an ideal choice for automating tasks, whether you're a seasoned programmer or a complete beginner.
What makes Python so user-friendly for automation?
Python's syntax is clean and intuitive, allowing users to write and understand code quickly. Unlike some other programming languages, Python prioritizes human readability, making it easier to learn and use. This is especially beneficial for beginners who want to dive into automation without getting bogged down by complex syntax.
How does Python's library ecosystem enhance automation?
One of Python's greatest strengths lies in its vast library ecosystem. Libraries such as:
Read also:Sigil Of Lucifer Symbol Of Enlightenment And Power
- os and shutil for file and directory management
- BeautifulSoup and Scrapy for web scraping
- smtplib and imaplib for email automation
- pandas and openpyxl for data analysis and spreadsheet processing
These libraries provide pre-built functionality, reducing the amount of code you need to write and enabling you to focus on solving problems rather than reinventing the wheel.
How to Get Started with Python?
Getting started with Python is easier than you might think. Here's a step-by-step guide to help you begin your automation journey:
- Install Python: Visit the official Python website (https://www.python.org) and download the latest version of Python for your operating system. Follow the installation instructions provided.
- Set Up a Code Editor: Choose a code editor or integrated development environment (IDE) such as Visual Studio Code, PyCharm, or Jupyter Notebook to write and test your Python scripts.
- Learn the Basics: Familiarize yourself with Python's syntax, data types, and control structures. Online platforms like Codecademy, freeCodeCamp, and Coursera offer excellent Python tutorials.
- Install Libraries: Use Python's package manager, pip, to install libraries and modules required for automation. For example, run
pip install pandas
to install the pandas library. - Start Small: Begin with simple automation tasks, such as renaming files or sending automated emails. Gradually move on to more complex projects as your skills improve.
Remember, practice makes perfect! The more you experiment and create, the more confident you'll become in automating tasks with Python.
Essential Python Concepts for Automation
Before diving into complex automation tasks, it's crucial to understand some fundamental Python concepts. These concepts form the backbone of any automation script:
- Variables and Data Types: Learn how to store and manipulate data using variables and understand Python's basic data types (e.g., strings, integers, floats, and lists).
- Control Structures: Master Python's if-else statements, loops (for and while), and conditional expressions to control the flow of your scripts.
- Functions: Understand how to define and call functions to organize your code and make it reusable.
- Modules and Libraries: Learn how to import and use Python's built-in and third-party libraries for various tasks.
- Error Handling: Familiarize yourself with Python's try-except blocks to handle errors gracefully and ensure your scripts run smoothly.
By mastering these concepts, you'll have a solid foundation to tackle any automation challenge with confidence.
What Tasks Can You Automate with Python?
Python's versatility makes it suitable for automating a wide range of tasks. Here are some common examples:
- File Management: Renaming, moving, or deleting files and folders based on specific criteria.
- Web Scraping: Extracting data from websites and saving it in a structured format (e.g., CSV or Excel).
- Email Automation: Sending and receiving automated emails, organizing inboxes, and filtering messages.
- Data Entry: Automating repetitive data entry tasks in spreadsheets or databases.
- Data Analysis: Processing and visualizing data using libraries like pandas and matplotlib.
The possibilities are virtually endless, and your creativity is the only limit!
Step-by-Step Guide to Automating Tasks
Automation can seem intimidating at first, but breaking it down into manageable steps makes it much more approachable. Follow these steps to automate a task with Python:
- Define the Task: Clearly identify the task you want to automate and its objectives.
- Research and Plan: Determine the libraries and tools you'll need and outline the steps to achieve your goal.
- Write the Script: Use Python to write a script that performs the task. Start simple and add complexity as needed.
- Test and Debug: Run your script and identify any errors or issues. Debug your code to ensure it works as intended.
- Optimize and Automate: Refactor your code to improve its efficiency and set up automation using task schedulers or cron jobs.
By following this process, you'll be able to tackle complex automation projects with ease.
Automate File Management Tasks
File management is one of the most common areas where Python automation shines. Tasks like organizing files, renaming them, or deleting duplicates can be done effortlessly with Python scripts. For instance:
import os directory = '/path/to/your/folder' for filename in os.listdir(directory): if filename.endswith('.txt'): new_name = filename.replace('old', 'new') os.rename(os.path.join(directory, filename), os.path.join(directory, new_name))
This script renames all text files in a folder, replacing "old" with "new" in their filenames. Simple yet powerful!
How to Automate Web Scraping Tasks?
Web scraping involves extracting data from websites and saving it for analysis or reporting. Python libraries like BeautifulSoup and Selenium make this process straightforward. Here's a basic example using BeautifulSoup:
from bs4 import BeautifulSoup import requests url = 'https://example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') for link in soup.find_all('a'): print(link.get('href'))
This script extracts and prints all the hyperlinks from a webpage. Remember to scrape responsibly and follow the website's terms of service!
Email Automation with Python
Python makes it easy to send and receive emails programmatically. Using the smtplib library, you can automate tasks like sending newsletters, reminders, or notifications. Here's an example:
import smtplib server = smtplib.SMTP('smtp.example.com', 587) server.starttls() server.login('your_email@example.com', 'your_password') message = 'Hello, this is an automated email!' server.sendmail('your_email@example.com', 'recipient@example.com', message) server.quit()
Replace the placeholders with your email credentials and customize the message as needed. Just like that, you've automated your emails!
Automate Data Entry and Analysis
Data entry and analysis are often repetitive and time-consuming tasks. Python's pandas library is a game-changer in this regard. Here's a simple example of automating data entry:
import pandas as pd data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Los Angeles', 'Chicago'] } df = pd.DataFrame(data) df.to_excel('output.xlsx', index=False)
This script creates a dataset and saves it as an Excel file. With pandas, you can also perform advanced data analysis, filtering, and visualization.
Advanced Tips for Python Automation
Once you've mastered the basics, you can take your automation skills to the next level with advanced techniques:
- Use regular expressions (regex) for pattern matching and text manipulation.
- Integrate Python with APIs to interact with external services and applications.
- Leverage multithreading and multiprocessing to improve the performance of your scripts.
- Set up Python automation scripts to run on a schedule using tools like Task Scheduler (Windows) or cron (Linux).
- Explore cloud-based automation solutions to scale your projects and collaborate with others.
These tips will help you tackle more complex projects and unlock the full potential of Python automation.
Common Errors and How to Fix Them
Automation scripts are not immune to errors. Here are some common issues you might encounter and how to resolve them:
- Syntax Errors: Double-check your code for typos, missing colons, or mismatched parentheses.
- Module Not Found: Ensure the required libraries are installed using pip.
- File Not Found: Verify file paths and ensure the file exists in the specified location.
- Permission Errors: Run your script with the necessary permissions or adjust file access settings.
- API Rate Limits: Implement delays or retries to avoid exceeding API usage limits.
By addressing these issues proactively, you can ensure your scripts run smoothly and efficiently.
Is Automate the Boring Stuff with Python Right for You?
Whether you're a student, a professional, or someone looking to improve their productivity, Automate the Boring Stuff with Python is a valuable resource. It equips you with the skills to streamline everyday tasks, enhance your efficiency, and even open doors to new career opportunities in programming and automation.
FAQs About Automate the Boring Stuff with Python
1. Do I need prior programming experience to use Automate the Boring Stuff with Python?
No, the book is designed for beginners and assumes no prior programming knowledge. It provides step-by-step instructions and practical examples to help you get started.
2. What tools do I need to follow along with the book?
You'll need a computer with Python installed and a code editor or IDE. The book also recommends installing additional libraries for specific tasks.
3. Can I use Automate the Boring Stuff with Python for professional projects?
Absolutely! The skills and techniques covered in the book are applicable to real-world projects and can be used in various professional settings.
4. What version of Python is used in the book?
The book primarily uses Python 3, so it's recommended to install the latest version of Python 3 to follow along.
5. Are there any prerequisites for learning Python automation?
Aside from basic computer literacy, there are no prerequisites. A willingness to learn and experiment is all you need!
6. How can I practice the concepts learned in the book?
Try automating tasks in your daily life, work, or studies. The more you practice, the more proficient you'll become.
Conclusion
Automate the Boring Stuff with Python is a game-changing resource for anyone looking to enhance their productivity and embrace the power of automation. By mastering Python and its vast array of libraries, you can simplify your daily routines, tackle complex tasks, and unlock new opportunities in the tech-driven world. So, why wait? Start automating today and experience the transformative impact of Python on your life!
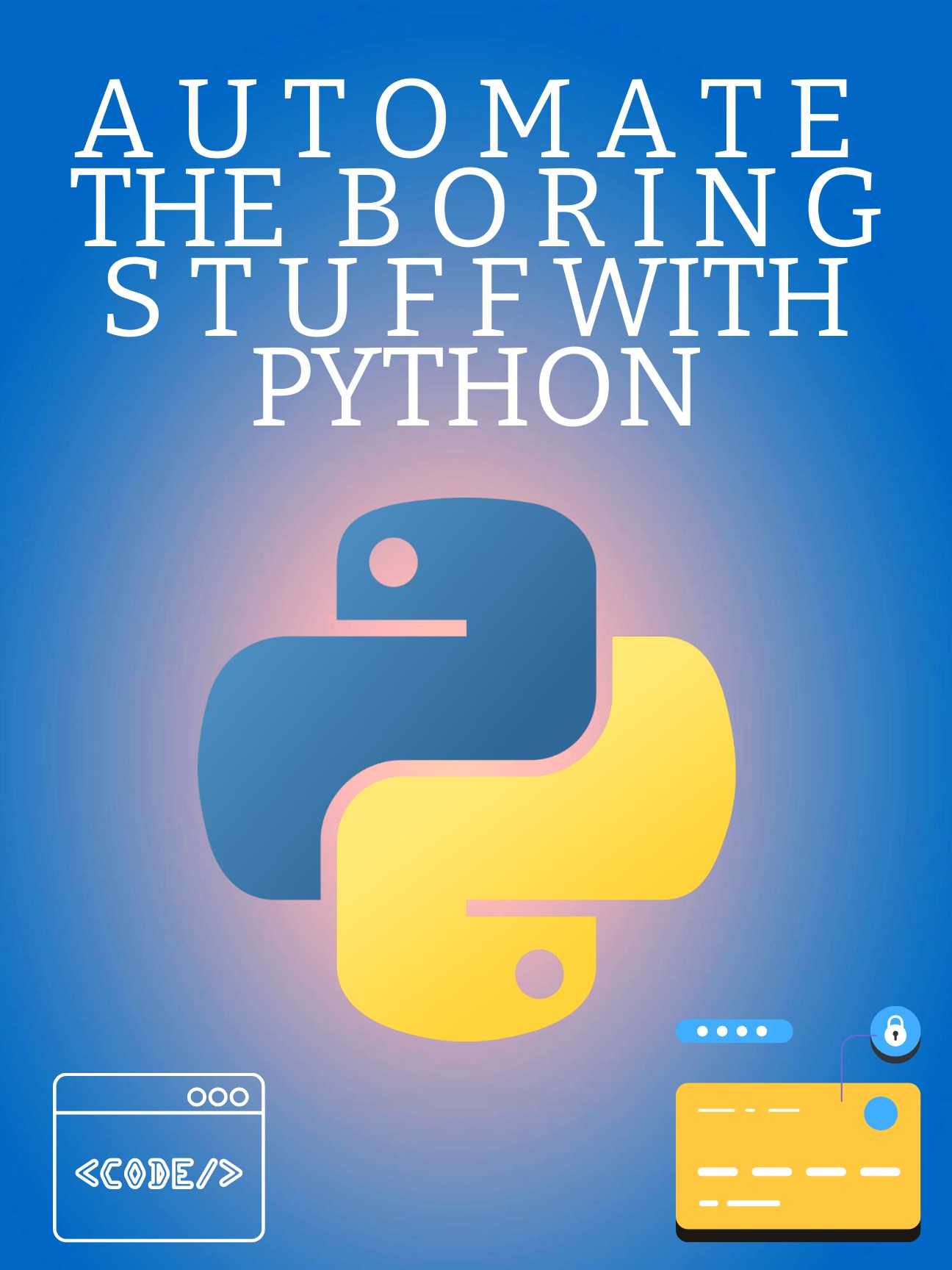
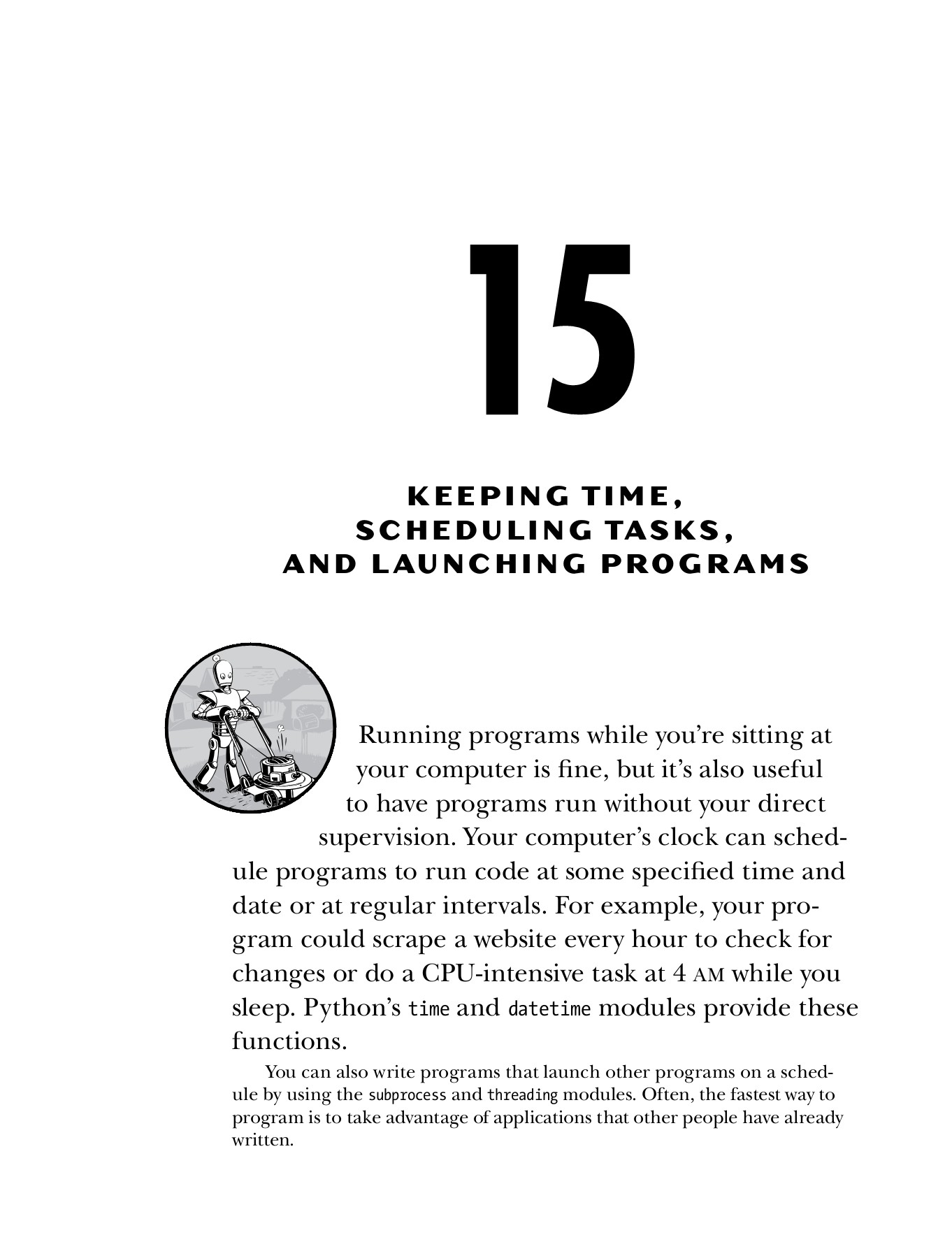