Python is a powerful programming language, known for its simplicity and versatility. While it provides built-in functions like sorting to help developers manage lists efficiently, there are scenarios where sorting a list might not be the best or most efficient option. This is especially true when you're only interested in retrieving a few of the smallest elements from a list without needing to rearrange the entire dataset. For such cases, Python offers several alternative approaches that are both practical and performance-optimized.
Whether you're a beginner or an experienced programmer, understanding how to get multiple smallest elements in a list without sorting Python can be incredibly beneficial. This skill can save computational resources, especially when dealing with large datasets. Moreover, it provides insights into Python's rich ecosystem of functions and libraries, enabling you to write cleaner and more efficient code. But how do you achieve this without relying on the traditional sort function? That’s exactly what we’ll be covering in this detailed guide.
In this article, we’ll explore various techniques to fetch multiple smallest elements from a list without sorting it. From using built-in Python functions like `heapq.nsmallest()` to implementing custom algorithms, we’ll break down each method step by step. Along the way, we’ll also address common challenges and pitfalls, ensuring that you have a clear understanding of how to apply these techniques in real-world scenarios. Let’s dive in!
Read also:Enhanced Home Aesthetics Atrium Windows And Doors
Table of Contents
- Why Avoid Sorting?
- How Does Python Handle Lists?
- What Are the Alternatives to Sorting?
- Using Python's Heapq Library
- Practical Example: Fetching Smallest Elements with Heapq
- Iterative Approach to Find Smallest Elements
- Why Is the Iterative Method Useful?
- Can We Use Min() and Remove() Multiple Times?
- Custom Functions for Specific Use Cases
- Why Does Efficiency Matter?
- Handling Edge Cases in List Operations
- Real-World Applications of These Techniques
- How to Handle Large Datasets?
- Comparison of Methods
- FAQs
- Conclusion
Why Avoid Sorting?
Sorting a list is one of the most common operations in Python. However, it might not always be the best choice, especially when dealing with large datasets. Sorting has a time complexity of O(n log n), which can be computationally expensive when you only need to access a few smallest elements. Why sort the entire list when you only care about a handful of values?
Here are some reasons to avoid sorting:
- Efficiency: Sorting rearranges the entire list, which is unnecessary if you're only interested in a few elements.
- Data Integrity: Sorting changes the order of elements, which might not be acceptable in certain cases.
- Memory Usage: Sorting can require additional memory, especially when working with large lists.
Understanding these challenges is the first step toward exploring alternative methods to get the smallest elements effectively. Let’s now delve into how Python handles lists and what makes them so versatile.
How Does Python Handle Lists?
Lists are one of the most versatile and frequently used data structures in Python. They allow you to store multiple items in a single variable, and their dynamic nature lets you modify them easily. Python lists are implemented as dynamic arrays, meaning their size can change as you add or remove elements.
Key characteristics of Python lists include:
- They are ordered and allow duplicate elements.
- They can store elements of different data types.
- They support various operations like indexing, slicing, and iteration.
Given these features, lists are often the go-to choice for managing collections of data. However, their flexibility also means that developers need to be cautious about performance, especially when working with large lists or performing computationally expensive operations like sorting.
Read also:Comprehensive Guide To Cyrus One A Leading Data Center Provider
What Are the Alternatives to Sorting?
When it comes to fetching multiple smallest elements from a list, sorting is not the only option. Python offers several alternative methods that are both efficient and easy to implement:
- Using the
heapq
library to retrieve the smallest elements. - Iteratively finding the smallest elements using the
min()
function. - Creating custom functions tailored to specific requirements.
Each of these methods has its pros and cons, which we’ll explore in detail in the following sections. For now, let’s focus on Python’s heapq
library, a built-in module that provides a simple way to work with heaps.
Using Python's Heapq Library
The heapq
module is part of Python’s standard library and provides an efficient way to perform heap operations. A heap is a specialized tree-based data structure that satisfies the heap property. In a min-heap, for example, the smallest element is always at the root.
Key functions in the heapq
module include:
heapify(iterable):
Converts an iterable into a heap.heappush(heap, element):
Adds an element to the heap.heappop(heap):
Removes and returns the smallest element.nsmallest(n, iterable):
Returns then
smallest elements from an iterable.
For our specific use case, the nsmallest()
function is particularly useful as it allows us to fetch multiple smallest elements efficiently without sorting the entire list.
Practical Example: Fetching Smallest Elements with Heapq
Here’s a step-by-step example of how to use the heapq
library to get multiple smallest elements from a list:
import heapq # Sample list numbers = [5, 2, 9, 1, 7, 3] # Get the 3 smallest elements smallest_elements = heapq.nsmallest(3, numbers) print(smallest_elements)
Output:
[1, 2, 3]
This method is both simple and efficient, making it a popular choice among developers. But what if you don’t want to use any external libraries? Let’s explore an iterative approach next.
Iterative Approach to Find Smallest Elements
If you prefer not to use the heapq
library, you can implement an iterative approach to fetch the smallest elements. This method involves repeatedly finding the smallest element in the list, appending it to a result list, and then removing it from the original list.
Here’s an example:
# Sample list numbers = [5, 2, 9, 1, 7, 3] # Number of smallest elements to fetch n = 3 # Result list smallest_elements = [] for i in range(n): smallest = min(numbers) smallest_elements.append(smallest) numbers.remove(smallest) print(smallest_elements)
While this method is straightforward, it’s not as efficient as using heapq
, especially for large lists. However, it’s a good alternative when external libraries are not an option.
Why Is the Iterative Method Useful?
The iterative method is particularly useful in scenarios where:
- You have limited resources and cannot use external libraries.
- You need to maintain control over the process, such as applying custom conditions or filters.
- You’re working with smaller datasets where performance is not a critical concern.
That said, it’s essential to weigh the pros and cons of each method to determine the best approach for your specific use case.
Can We Use Min() and Remove() Multiple Times?
Using the min()
function and remove()
method multiple times is a common approach to fetch the smallest elements from a list. However, this method has its limitations:
- Performance: Both
min()
andremove()
have a time complexity of O(n), making this approach less efficient for large lists. - Mutability: The original list is modified during the process, which might not be desirable in some cases.
If performance and data integrity are not major concerns, this approach can be a quick and easy solution. Otherwise, consider using alternatives like heapq
or custom functions.
Custom Functions for Specific Use Cases
In some scenarios, you might need to create custom functions to fetch the smallest elements. These functions can be tailored to meet specific requirements, such as handling unique constraints or optimizing performance.
For example, you could write a function that uses a priority queue or a binary search tree to efficiently retrieve the smallest elements. While these methods require more effort to implement, they can offer significant performance benefits for large datasets.
Why Does Efficiency Matter?
Efficiency is a critical factor when working with large datasets or applications that require real-time processing. Using an inefficient method to fetch the smallest elements can lead to performance bottlenecks, affecting the overall user experience.
By understanding the trade-offs of each method, you can make informed decisions that balance performance, simplicity, and resource usage. In the next section, we’ll discuss how to handle edge cases when working with lists.
Handling Edge Cases in List Operations
When working with lists, it’s essential to consider edge cases to ensure your code is robust and error-free. Some common edge cases include:
- Empty lists: Ensure your code can handle scenarios where the list is empty.
- Duplicate elements: Decide whether duplicates should be included in the results.
- Invalid input: Validate inputs to prevent errors or unexpected behavior.
By accounting for these edge cases, you can create more reliable and user-friendly applications.
Real-World Applications of These Techniques
Fetching the smallest elements from a list has numerous real-world applications, including:
- Data analysis and statistics.
- Prioritizing tasks or events.
- Optimizing algorithms and systems.
Understanding these applications can help you appreciate the importance of mastering these techniques. Let’s now explore how to handle large datasets effectively.
How to Handle Large Datasets?
When dealing with large datasets, efficiency becomes even more critical. Here are some tips to optimize performance:
- Use efficient algorithms like heaps or priority queues.
- Minimize memory usage by working with iterators or generators.
- Leverage Python’s built-in libraries for optimized performance.
By following these tips, you can handle large datasets more effectively and ensure your applications run smoothly.
Comparison of Methods
Here’s a quick comparison of the methods discussed:
Method | Efficiency | Ease of Use | Recommended For |
---|---|---|---|
Heapq | High | Easy | Most use cases |
Iterative | Medium | Moderate | Smaller datasets |
Custom Functions | Varies | Complex | Specific requirements |
FAQs
Here are some frequently asked questions about fetching the smallest elements from a list:
- Can I use a for loop to get the smallest elements? Yes, but it may not be the most efficient method.
- What happens if the list is empty? Most methods will raise an error, so it’s important to handle this case.
- Can I fetch the smallest elements without modifying the original list? Yes, methods like
heapq.nsmallest()
don’t modify the original list. - Is it possible to fetch elements based on custom conditions? Yes, you can use custom functions or filters to achieve this.
- How do I handle duplicate elements? Decide whether duplicates should be included in the results and implement your code accordingly.
- Are these methods suitable for real-time applications? Yes, methods like
heapq
are efficient enough for real-time use cases.
Conclusion
Understanding how to get multiple smallest elements in a list without sorting Python is a valuable skill that can enhance your programming expertise. By exploring methods like heapq
, iterative approaches, and custom functions, you can tackle this problem efficiently and effectively. Whether you’re working with small datasets or large-scale applications, these techniques provide the tools you need to optimize performance and achieve your goals. Now it’s your turn to apply these methods in your projects and see the results for yourself!
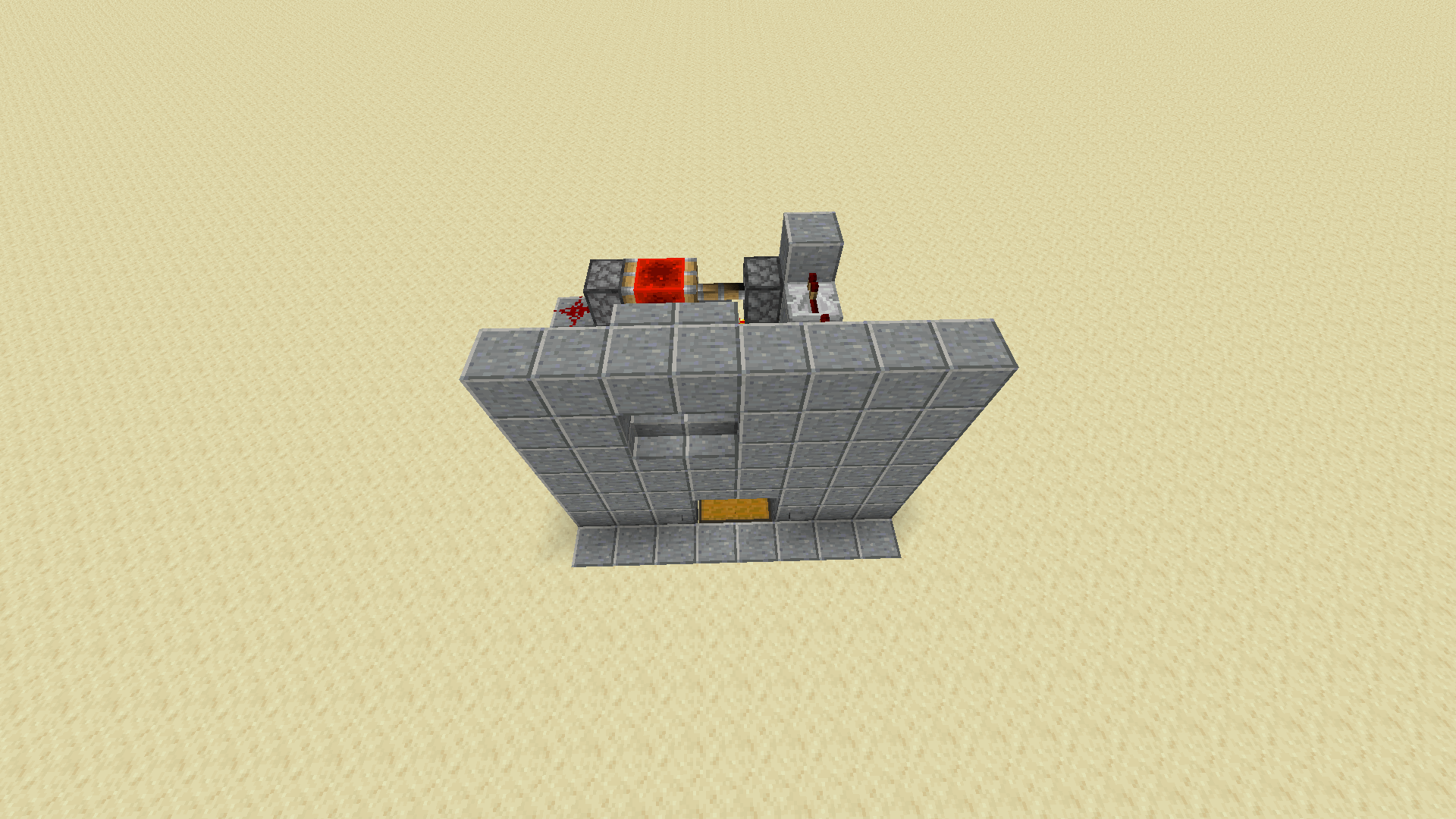
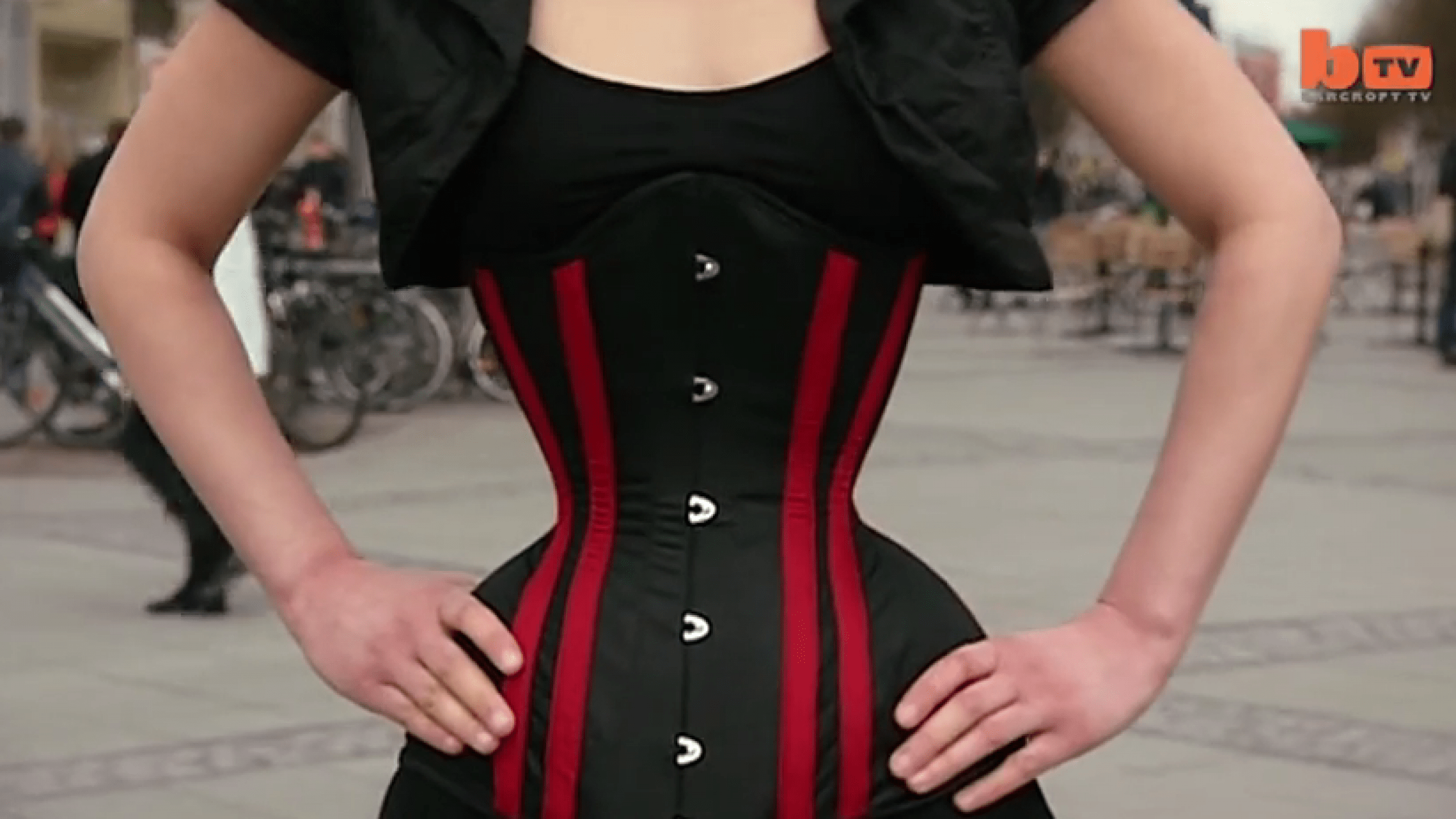